Virtual DOM vs Real DOM: Why Frontend Devs Care So Much
And how it affects app speed, user experience, and rendering.
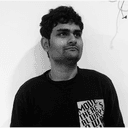
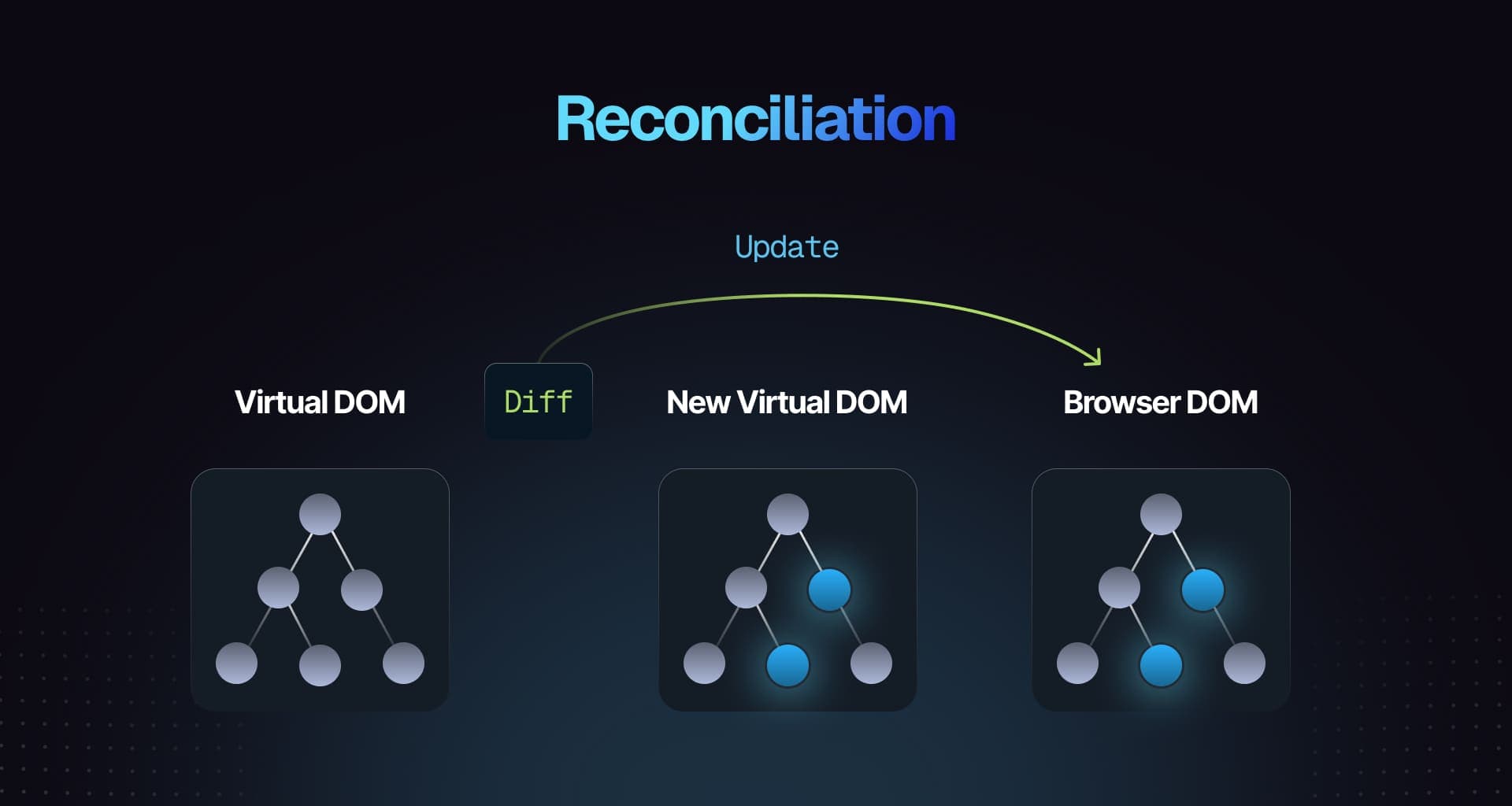
As a frontend developer in 2025, few architectural decisions impact your application's performance more than how you interact with the Document Object Model (DOM). The debate between Virtual DOM and direct DOM manipulation continues to shape framework choices, rendering strategies, and ultimately user experience. Let's dive deep into why this seemingly technical implementation detail generates such passionate discussion among developers.
🌳 Understanding the DOM: The Browser's Render Tree
Before we can appreciate the virtual vs. real DOM debate, we need to understand what the DOM actually is and why interacting with it can be problematic.
The Document Object Model represents your HTML as a tree structure where each element (div, span, p, etc.) is a node. This tree serves as the bridge between your JavaScript code and what users see in the browser. When users interact with your application, the DOM needs to update to reflect those changes.
The Challenge With Direct DOM Manipulation
The real DOM has been the foundation of web development since the beginning, but it comes with inherent challenges:
- 🐢 Performance bottlenecks: DOM operations are significantly slower than standard JavaScript operations
- 🔄 Reflow and repaint: Changing the DOM triggers expensive browser rendering processes
- 🧩 Complex state management: Tracking which parts of the DOM need updating becomes exponentially harder as applications grow
- 📱 Device limitations: On lower-powered devices, heavy DOM manipulation can lead to janky experiences
When developers directly manipulate the DOM (as in traditional jQuery-style development), each operation can trigger multiple browser recalculations. A simple update might cause the browser to recalculate styles, perform layout operations, paint pixels, and composite layers—all potentially blocking the main thread and causing performance issues.
🔄 Enter the Virtual DOM: An Abstraction Layer
The Virtual DOM emerged as a solution to these performance challenges, popularized by React and later adopted by numerous other frameworks. But what exactly is it?
The Virtual DOM is a lightweight JavaScript representation of the real DOM tree kept in memory. Think of it as a blueprint that can be modified freely without the performance costs of touching the actual browser rendering engine.
How the Virtual DOM Works
- Your application maintains a virtual representation of the DOM in memory
- When state changes occur, a new virtual DOM is created reflecting the changes
- This new virtual DOM is compared with the previous version (a process called "diffing")
- Only the differences ("diffs") are calculated
- The real DOM is updated only with the necessary changes, in an optimized batch
This approach minimizes direct DOM manipulation, reducing the expensive reflow and repaint operations that browsers must perform. The result is better performance and smoother user experiences, especially in dynamic applications with frequent updates.
⚔️ The Great Debate: Virtual DOM vs. Direct DOM Manipulation
Let's compare these approaches across several key dimensions:
Aspect | Virtual DOM | Direct DOM Manipulation |
---|---|---|
Performance for small changes | Slightly slower | Potentially faster |
Performance for multiple changes | Much faster | Significantly slower |
Memory usage | Higher (keeps copies in memory) | Lower |
Development ergonomics | Simpler, declarative | More complex, imperative |
State synchronization | Automatic | Manual |
Framework examples | React, Vue, Preact | Vanilla JS, jQuery, Alpine.js |
Learning curve | Conceptual understanding required | More straightforward initially |
Performance Implications: It's Complicated
The conventional wisdom that "Virtual DOM is always faster" isn't entirely accurate. The truth is more nuanced:
For small, isolated updates to the DOM, direct manipulation can actually be faster since it avoids the overhead of virtual DOM diffing and reconciliation. When you know exactly what needs to change, direct DOM manipulation is highly efficient.
For complex applications with frequent updates, the Virtual DOM's batching and minimization of actual DOM operations provide significant performance benefits. The ability to calculate the minimal set of changes needed is where Virtual DOM truly shines.
As one developer memorably put it: "Using Virtual DOM is like buying in bulk—there's some overhead, but it becomes more efficient as your needs scale up."
📊 Real-World Impact on User Experience
The technical differences between Virtual DOM and direct DOM manipulation translate to tangible effects on user experience:
🚀 Perceived Performance
Virtual DOM implementations often feel more responsive because they:
- Batch DOM updates to prevent partial renders
- Reduce main thread blocking
- Minimize visual "jank" during complex state transitions
- Allow for animation optimizations through keyed elements
These benefits are particularly noticeable on:
- Content-heavy pages with frequent updates
- Data visualization applications
- Real-time collaborative tools
- Complex form interfaces
🧠 Developer Experience and Mental Model
Beyond performance, the Virtual DOM provides a simpler mental model for developers:
- Declarative vs Imperative: With Virtual DOM, developers describe what the UI should look like based on current state, rather than manually orchestrating DOM changes
- State Synchronization: Less risk of UI and state becoming desynchronized
- Component Abstraction: Enables thinking in components rather than DOM operations
- Testing: Easier to test since components can be rendered virtually without a browser
This developer experience advantage often leads to fewer bugs and more maintainable code in complex applications.
🔍 When Virtual DOM Makes Sense (And When It Doesn't)
The Virtual DOM isn't always the right choice. Here's when each approach shines:
Virtual DOM Excels For:
- 📱 Complex, state-driven UIs: Applications with numerous interactive elements and frequent state changes
- 🔄 Component-based architecture: When your application is composed of reusable components
- 👥 Team development: Larger teams benefit from the more predictable programming model
- 🛠️ Complex state management: Applications where UI is derived from complex application state
- 🔄 High-frequency updates: UIs that update multiple times per second
Direct DOM Manipulation Shines For:
- 🪶 Lightweight interactions: Simple websites with minimal interactivity
- ⚡ Performance-critical animations: Custom animations requiring precise control
- 🧮 Small, focused utilities: When you need a small JS footprint
- 🎯 Targeted optimizations: Sometimes surgical DOM updates can boost performance in specific scenarios
- 📉 Low-memory environments: When memory constraints are significant
🧩 Modern Approaches: Beyond the Binary Choice
In 2025, the landscape has evolved beyond the simple Virtual DOM vs. Real DOM dichotomy. Modern frameworks have developed sophisticated approaches:
1️⃣ Incremental DOM (Google's Approach)
Unlike Virtual DOM which creates a complete tree in memory, Incremental DOM updates the existing DOM in place. This trades some performance in exchange for lower memory usage. Angular uses this approach for its rendering engine.
2️⃣ Svelte's Compile-Time Approach
Svelte shifts the work from runtime to compile time. Instead of using a Virtual DOM, Svelte compiles components into highly optimized JavaScript that directly updates the DOM. This approach provides the benefits of component-based development without the Virtual DOM overhead.
3️⃣ Solid.js's Fine-Grained Reactivity
Solid.js uses a reactive system that tracks dependencies at a granular level, allowing it to update only what has actually changed without diffing. This approach offers Virtual DOM-like developer experience with direct DOM manipulation performance.
4️⃣ React Server Components
React Server Components represent a hybrid approach where some components render on the server with their output streamed to the client, reducing the JavaScript shipped to users while maintaining the Virtual DOM model for interactive elements.
📝 Code Comparison: Same Feature, Different Approaches
To illustrate the differences, let's look at implementing a simple counter in both paradigms:
Virtual DOM Approach (React):
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
Direct DOM Approach (Vanilla JS):
// Create elements
const container = document.createElement("div");
const paragraph = document.createElement("p");
const button = document.createElement("button");
// Set initial state
let count = 0;
paragraph.textContent = `Count: ${count}`;
button.textContent = "Increment";
// Add event listener
button.addEventListener("click", () => {
count++;
paragraph.textContent = `Count: ${count}`;
});
// Compose DOM structure
container.appendChild(paragraph);
container.appendChild(button);
document.body.appendChild(container);
The Virtual DOM approach is more declarative and focuses on what the UI should look like at any given state. The direct DOM approach requires manually orchestrating each DOM update.
🔬 Performance Benchmarks: What the Data Says
Recent benchmarking data gives us insight into how different approaches perform:
Framework/Approach | Initial Render | Memory Usage | Update Speed | Bundle Size |
---|---|---|---|---|
React (Virtual DOM) | Moderate | Higher | Fast for complex updates | Larger |
Vue (Virtual DOM) | Moderate | Moderate | Fast for complex updates | Moderate |
Svelte (Compile-time) | Fast | Low | Very fast | Smallest |
Vanilla JS (Direct DOM) | Fastest | Lowest | Fast for simple updates | Minimal |
Solid.js (Fine-grained) | Fast | Low | Fastest | Small |
These benchmarks highlight why there's no universal "best" approach—each has different strengths depending on the specific metrics you prioritize.
🧪 Making the Right Choice for Your Project
When deciding between Virtual DOM and direct DOM manipulation (or one of the hybrid approaches), consider:
- Application complexity: How dynamic is your UI? How many components interact with each other?
- Performance requirements: What are your target devices? Mobile? High-end desktops?
- Team familiarity: What is your team most comfortable with?
- Long-term maintenance: Who will maintain this code in the future?
- Bundle size constraints: How important is initial load performance?
Most importantly, remember that these technologies are tools, not identities. The best developers understand the tradeoffs and choose the right approach for each specific project rather than becoming ideologically attached to one paradigm.
📈 The Future: Where DOM Manipulation Is Heading
Looking forward, several trends are emerging:
- Island Architecture: Combining static content with islands of interactivity
- Resumable frameworks: Hydrating only the interactive parts of an application
- Server Components: Moving more rendering to the server while maintaining interactivity
- Web Components: Standardized component model with better browser integration
- Fine-grained reactivity: More precise updates without full Virtual DOM overhead
The line between Virtual DOM and direct manipulation approaches continues to blur as frameworks adopt the best aspects of each approach.
🏁 Conclusion: Choose Your Tool Wisely
The Virtual DOM vs. Real DOM debate reveals a fundamental truth about frontend development: there are always tradeoffs. Virtual DOM offers a simpler programming model and efficient updates for complex applications at the cost of additional abstraction and memory usage. Direct DOM manipulation provides maximum control and potentially better performance for simpler interactions.
The most successful frontend developers understand these tradeoffs rather than dogmatically adhering to one approach. By understanding the strengths and weaknesses of each pattern, you can make informed decisions that balance performance, developer experience, and user experience.
As your applications grow in complexity, the benefits of Virtual DOM and component-based approaches often outweigh the performance advantages of direct manipulation. However, for performance-critical sections or simple applications, direct DOM updates remain a powerful tool in your arsenal.
What's your experience with these different rendering approaches? Have you found particular use cases where one clearly outperforms the other? The conversation continues!